TIL (Today I Learned)
A blog about web development, HTML, CSS, JavaScript, and web accessibility.
TIL #49
You can style the file selector button of file upload elements.
<label for="upload">Select file</label><br>
<input type="file" id="upload">
[type="file"]::file-selector-button {
background-color: hotpink;
padding: 0.5rem 1rem;
border: 2px solid fuchsia;
margin-block-end: 1rem;
display: block;
border-radius: 3px;
}
[type="file"]::file-selector-button:hover {
background-color: aqua;
}
TIL #48
You can use the :scope pseudo class to select direct children of an element with `.querySelectorAll()`.
<ul>
<li>A</li>
<li>B</li>
<li>C</li>
</ul>
<p>D</p>
console.log(document.body.querySelectorAll('*'))
// NodeList(5) [ul, li, li, li, p]
console.log(document.body.querySelectorAll(':scope > *'))
// NodeList(2) [ul, p]
TIL #47
console.count() logs the number of times that this particular call to count() has been called.
const letters = "availabilities".split("");
letters.forEach(letter => {
if (letter === 'i') {
console.count(`Letter ${letter}`)
}
})
/* Output:
Letter i: 1
Letter i: 2
Letter i: 3
Letter i: 4
*/
TIL #46
You can link to multiple e-mail addresses
<!-- comma separated list without spaces and/or url encoded with %20 -->
<a href="mailto:manuel@matuzo.at,manuel@webclerks.at">
Contact me and me
</a>
<!-- or with ?to= parameter -->
<a href="mailto:manuel@matuzo.at?to=manuel@webclerks.at">
Contact me and me
</a>
TIL #45
You can use the spellcheck attribute to instruct browsers that, if possible, an element should or should not be checked for spelling errors.
<textarea spellcheck="false">
Tis is jusst a test.
</textarea>
<div contenteditable spellcheck="false">
Tis is jusst a test.
</div>
TIL #44
You can animate z-index.
div {
position: absolute;
top: 0;
left: 0;
transition: z-index 2.5s;
}
TIL #43
You can make a link in an iframe open in its parent window, if you set target="_parent".
<!-- Parent File-->
<iframe src="https://codepen.io/matuzo/debug/YzGVXGV" frameborder="0"></iframe>
<!-- Embedded file -->
<ul>
<li>
<a href="https://a11yproject.com">no target</a>
</li>
<li>
<a href="https://a11yproject.com" target="_blank">target="_blank"</a>
</li>
<li>
<a href="https://a11yproject.com" target="_parent">target="_parent"</a>
</li>
</ul>
TIL #42
Adding an i (or I) before the closing bracket in an attribute selector causes the value to be compared case-insensitively.
<button class="mybutton">Send</button> <!-- red border -->
<button class="myButton">Send</button> <!-- green border -->
[class*="button" i] { /* matches mybutton and myButton */
border: 10px solid green;
}
[class*="button"] { /* matches only mybutton */
border-color: red;
}
TIL #41
ol and ul accept the type attribute. You can use it to set a different numbering type in HTML.
<ol type="a">
<li>Element 1</li>
<li>Element 2</li>
<li>Element 3</li>
</ol>
<ol type="A">
<li>Element 1</li>
<li>Element 2</li>
<li>Element 3</li>
</ol>
<ol type="i">
<li>Element 1</li>
<li>Element 2</li>
<li>Element 3</li>
</ol>
<ol type="I">
<li>Element 1</li>
<li>Element 2</li>
<li>Element 3</li>
</ol>
<ul type="1">
<li>Element 1</li>
<li>Element 2</li>
<li>Element 3</li>
</ul>
TIL #40
The order in which transform functions in the transform shorthand property are defined matters.
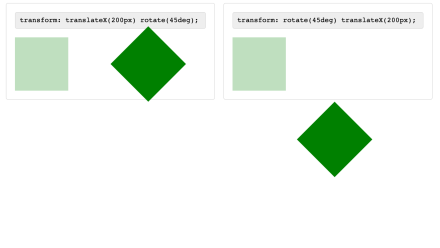
TIL #39
You can use the nomodule attribute to run JavaScript code only in browsers that don’t support JS modules.
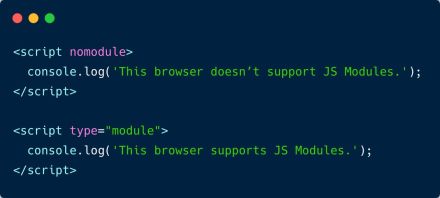
TIL #38
Using the background-origin property you can position background images relative to the inner border edge (default), outer border edge, or the content edge.
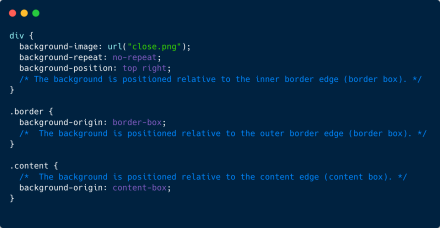
TIL #37
You can add the option { once: true } to an event listener to automatically remove it when has been invoked
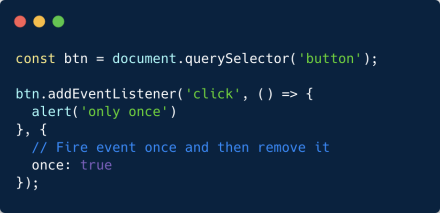
TIL #36
The nullish coalescing operator (??) only returns its right-hand side operand when its left-hand side operand is null or undefined.
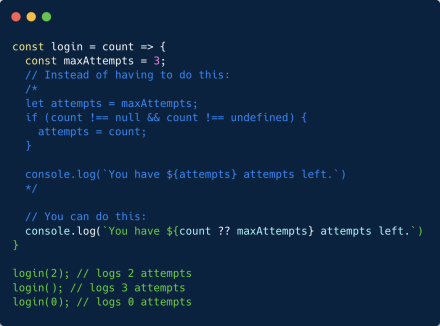
TIL #35
Optional chaining allows you to chain methods or properties, and conditionally continue down the chain if the value is not null or undefined.
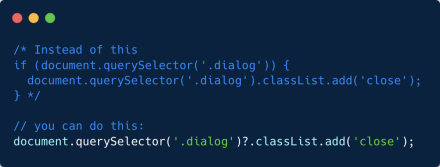
TIL #34
You can use the removeProperty method to remove a specific inline CSS property defined in the style attribute.

TIL #33
There’s a space-separated syntax for values in functional color notations.
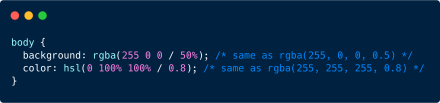
TIL #32
JSON.stringify takes 3 parameters. The third parameter can be used to pretty-print the output.
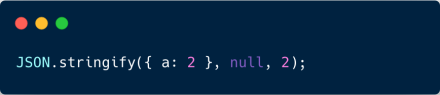
TIL #31
There's native, CSS-only smooth scrolling.
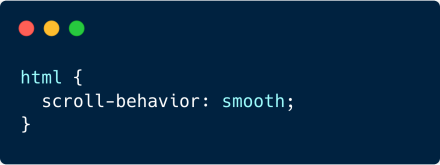
TIL #30
You can target Inverted Colors Mode mode in CSS
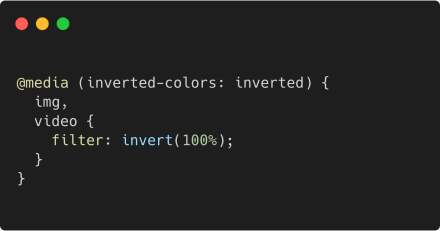
TIL #29
You can use JavaScript (Vibration API) to vibrate devices
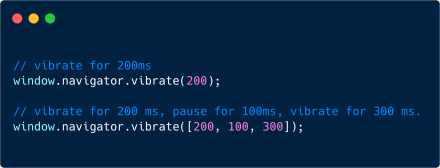
TIL #28
You can disable all form elements in a fieldset by setting the disabled attribute on the fieldset.
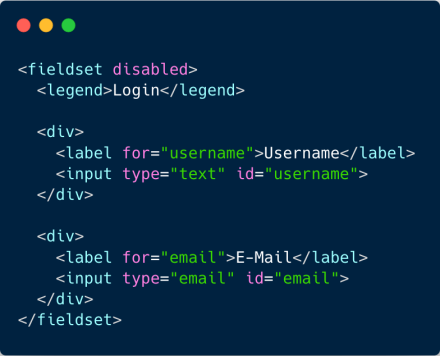
TIL #27
You can list all pseudo elements of an element in the CSS pane in Firefox Developer Tools.
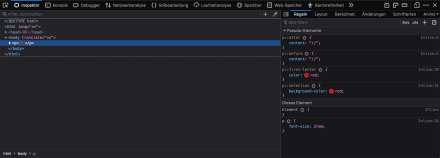
TIL #26
You can emulate dark mode and reduced motion in Chrome Dev Tools.
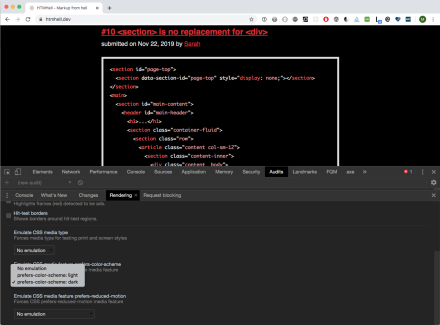
TIL #25
You can instruct browsers to print pages in landscape orientation (Chrome/Opera only).
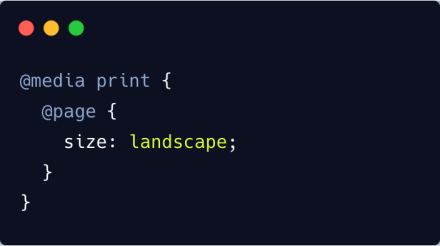
TIL #24
You can change the filename of a downloadable file by defining a value in the download attribute.
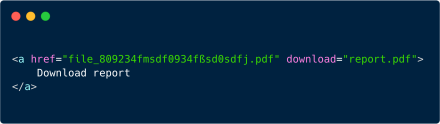
TIL #23
The bullet or number of a list-item can be selected and styled with the ::marker pseudo-element.
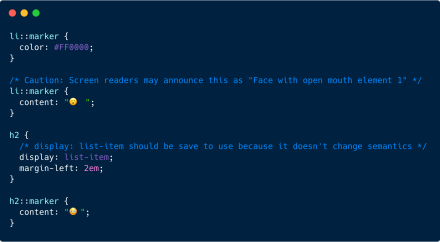
TIL #22
If an input element has the attribute value autocomplete="new-password", browsers can suggest securely-generated passwords.
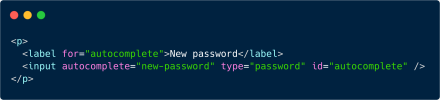
TIL #21
You can use the CSS Paint API to create background images in CSS.
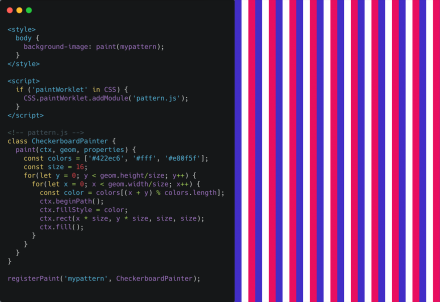
TIL #20
You can search the elements panel in Developer Tools not only for strings but also selectors.
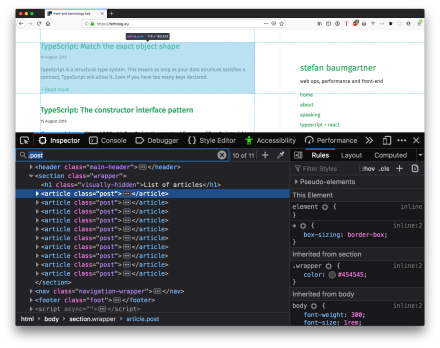
TIL #19
The webkitdirectory attribute allows users to select directories instead of files. (desktop browsers only)
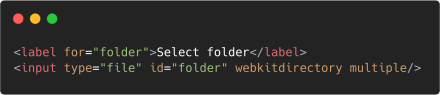
TIL #18
It's possible to identify how many separate fingers are touching the screen.
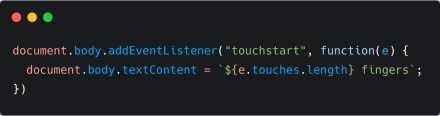
TIL #17
You can do responsive preloading because preload link elements accept the media attribute like any other link element.

TIL #16
SVGs are focusable in Internet Explorer. We can set focusable="false" to prevent that.
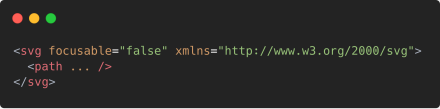
TIL #15
macOS uses Papyrus as the default font when you define the generic font family “fantasy”.
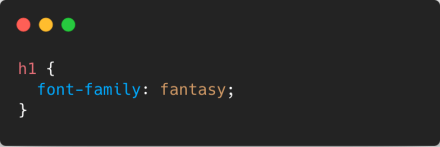
TIL #14
text-decoration doesn't have to be a solid line. There’s also dotted, dashed, double, and wavy.
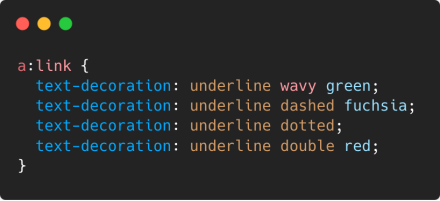
TIL #13
text-decoration is a shorthand property for text-decoration-line, text-decoration-color, and text-decoration-style.
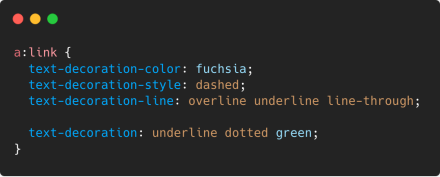
TIL #12
Overflowing block-level elements with overflow set to scroll or auto are focusable in Firefox.
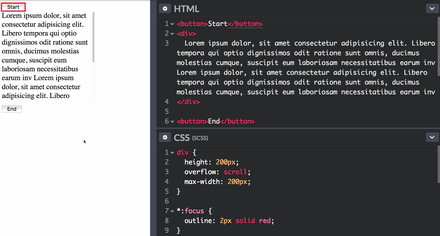
TIL #11
You can use conditional comments to target specific versions of Outlook.
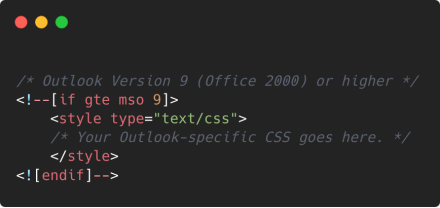
TIL #10
You can turn the outline of an element to the _inside_ by setting the outline-offset property to a negative value. (Not supported in IE)
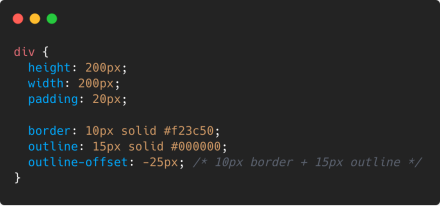
TIL #9
You can center a flex-item vertically and horizontally by applying margin: auto; to it.
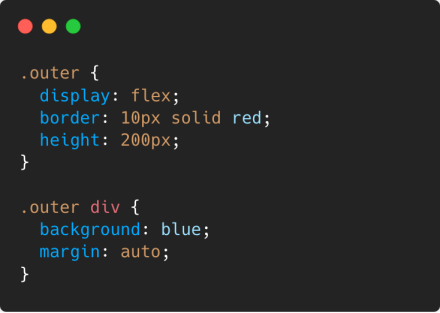
TIL #8
If you press CMD/Ctrl and click on a property or property value in the style panel, @ChromeDevTools jumps directly to the respective css/scss file and line.
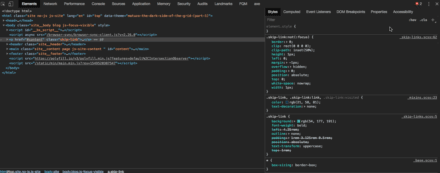
TIL #7
About the @at-root directive. It moves nested styles out from within a parent selector or nested directive.
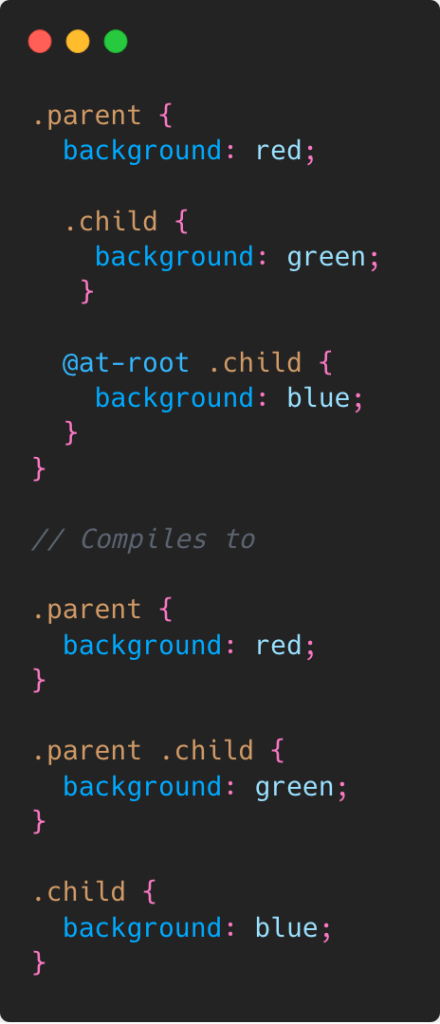
TIL #6
IE 11 requires a unit to be added to the third argument (flex-basis) of the flex property.
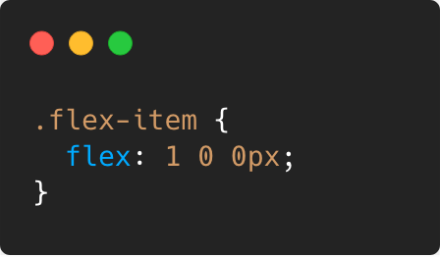
TIL #5
You can use `navigator.connection` to get information about the connection like round-trip time, bandwidth, connection type (e.g. 3g, 4g) or if Data-Saver is enabled.
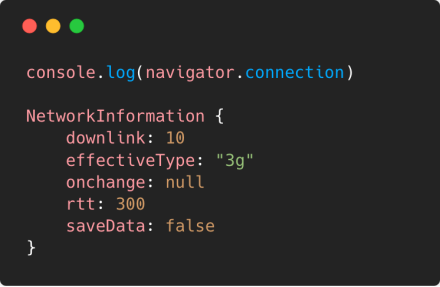
TIL #4
The grid-auto-flow property takes up to two values. row, column, dense, row dense, or column dense.
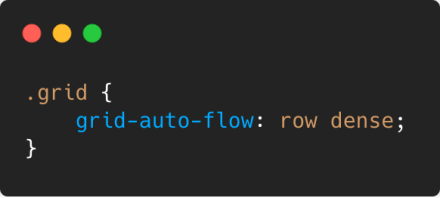
TIL #3
You can pass an `options` object, which only has a single property, to the `focus()` method to prevent scrolling on focus.
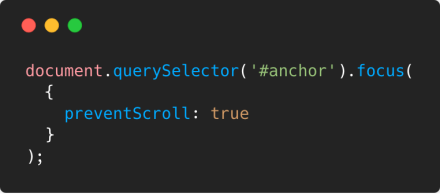
TIL #2
There’s a tab-size property. It controls the width of the tab (U+0009) character.
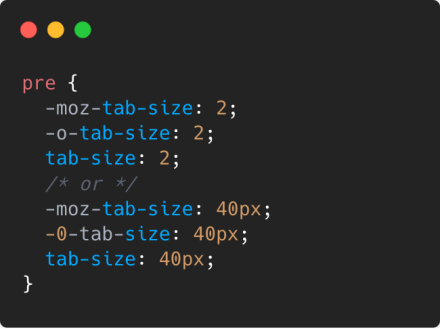
TIL #1
How to get a random background-color (or any other value) each time I compile Sass.
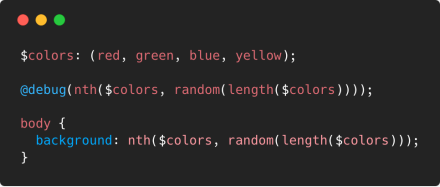